PhysiologyEngineThunk Class Referenceabstract
#include <PhysiologyEngineThunk.h>
Inheritance diagram for PhysiologyEngineThunk:
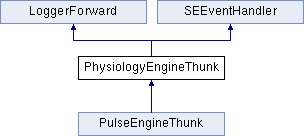
Public Member Functions | |
PhysiologyEngineThunk (const std::string &dataDir="./") | |
virtual | ~PhysiologyEngineThunk () |
virtual void | Clear () |
bool | SerializeFromFile (std::string const &filename, std::string const &data_requests, eSerializationFormat data_requests_format) |
bool | SerializeToFile (std::string const &filename) |
bool | SerializeFromString (std::string const &state, std::string const &data_requests, eSerializationFormat format) |
std::string | SerializeToString (eSerializationFormat format) |
bool | InitializeEngine (std::string const &patient_configuration, std::string const &data_requests, eSerializationFormat format) |
std::string | GetInitialPatient (eSerializationFormat format) |
std::string | GetConditions (eSerializationFormat format) |
void | LogToConsole (bool b) |
void | KeepLogMessages (bool keep) |
void | SetLogFilename (std::string const &logfile) |
std::string | PullLogMessages (eSerializationFormat format) |
void | SetLoggerForward (LoggerForward *lf) |
void | KeepEventChanges (bool keep) |
std::string | PullEvents (eSerializationFormat format) |
std::string | PullActiveEvents (eSerializationFormat format) |
std::string | GetPatientAssessment (int type, eSerializationFormat format) |
void | SetEventHandler (SEEventHandler *eh) |
bool | ProcessActions (std::string const &actions, eSerializationFormat format) |
std::string | PullActiveActions (eSerializationFormat format) |
bool | AdvanceTimeStep () |
double | GetTimeStep (std::string const &unit) |
size_t | DataLength () const |
double * | PullDataPtr () |
void | PullData (std::vector< double > &data) |
void | ForwardDebug (const std::string &msg) override |
void | ForwardInfo (const std::string &msg) override |
void | ForwardWarning (const std::string &msg) override |
void | ForwardError (const std::string &msg) override |
void | ForwardFatal (const std::string &msg) override |
void | HandleEvent (eEvent type, bool active, const SEScalarTime *time=nullptr) override |
![]() | |
virtual | ~LoggerForward ()=default |
![]() | |
SEEventHandler () | |
virtual | ~SEEventHandler () |
Protected Member Functions | |
virtual void | AllocateEngine ()=0 |
virtual void | SetupDefaultDataRequests () |
virtual bool | SetupRequests () |
Protected Attributes | |
std::unique_ptr< PhysiologyEngine > | m_engine |
SESubstanceManager * | m_subMgr = nullptr |
bool | m_keepLogMsgs = false |
bool | m_keepEventChanges = false |
LoggerForward * | m_ForwardLogs =nullptr |
SEEventHandler * | m_ForwardEvents =nullptr |
LogMessages | m_logMsgs |
std::string | m_dataDir ="./" |
std::vector< const SEEventChange * > | m_events |
std::vector< const SEActiveEvent * > | m_activeEvents |
size_t | m_length =0 |
double * | m_requestedData = nullptr |
std::vector< double > | m_requestedValues |
Detailed Description
An instance of an engine where the interface is define in stl and base data types. This interface is a thunk layer using serialized cdm objects to drive a Pulse engine.
Constructor & Destructor Documentation
◆ PhysiologyEngineThunk()
PhysiologyEngineThunk::PhysiologyEngineThunk | ( | const std::string & | dataDir = "./" | ) |
◆ ~PhysiologyEngineThunk()
|
virtual |
Member Function Documentation
◆ AdvanceTimeStep()
bool PhysiologyEngineThunk::AdvanceTimeStep | ( | ) |
◆ AllocateEngine()
|
protectedpure virtual |
Implemented in PulseEngineThunk.
◆ Clear()
|
virtual |
◆ DataLength()
size_t PhysiologyEngineThunk::DataLength | ( | ) | const |
◆ ForwardDebug()
|
overridevirtual |
Reimplemented from LoggerForward.
◆ ForwardError()
|
overridevirtual |
Reimplemented from LoggerForward.
◆ ForwardFatal()
|
overridevirtual |
Reimplemented from LoggerForward.
◆ ForwardInfo()
|
overridevirtual |
Reimplemented from LoggerForward.
◆ ForwardWarning()
|
overridevirtual |
Reimplemented from LoggerForward.
◆ GetConditions()
std::string PhysiologyEngineThunk::GetConditions | ( | eSerializationFormat | format | ) |
◆ GetInitialPatient()
std::string PhysiologyEngineThunk::GetInitialPatient | ( | eSerializationFormat | format | ) |
◆ GetPatientAssessment()
std::string PhysiologyEngineThunk::GetPatientAssessment | ( | int | type, |
eSerializationFormat | format | ||
) |
◆ GetTimeStep()
double PhysiologyEngineThunk::GetTimeStep | ( | std::string const & | unit | ) |
◆ HandleEvent()
|
overridevirtual |
Implements SEEventHandler.
◆ InitializeEngine()
bool PhysiologyEngineThunk::InitializeEngine | ( | std::string const & | patient_configuration, |
std::string const & | data_requests, | ||
eSerializationFormat | format | ||
) |
◆ KeepEventChanges()
void PhysiologyEngineThunk::KeepEventChanges | ( | bool | keep | ) |
◆ KeepLogMessages()
void PhysiologyEngineThunk::KeepLogMessages | ( | bool | keep | ) |
◆ LogToConsole()
void PhysiologyEngineThunk::LogToConsole | ( | bool | b | ) |
◆ ProcessActions()
bool PhysiologyEngineThunk::ProcessActions | ( | std::string const & | actions, |
eSerializationFormat | format | ||
) |
◆ PullActiveActions()
std::string PhysiologyEngineThunk::PullActiveActions | ( | eSerializationFormat | format | ) |
◆ PullActiveEvents()
std::string PhysiologyEngineThunk::PullActiveEvents | ( | eSerializationFormat | format | ) |
◆ PullData()
void PhysiologyEngineThunk::PullData | ( | std::vector< double > & | data | ) |
◆ PullDataPtr()
double * PhysiologyEngineThunk::PullDataPtr | ( | ) |
◆ PullEvents()
std::string PhysiologyEngineThunk::PullEvents | ( | eSerializationFormat | format | ) |
◆ PullLogMessages()
std::string PhysiologyEngineThunk::PullLogMessages | ( | eSerializationFormat | format | ) |
◆ SerializeFromFile()
bool PhysiologyEngineThunk::SerializeFromFile | ( | std::string const & | filename, |
std::string const & | data_requests, | ||
eSerializationFormat | data_requests_format | ||
) |
◆ SerializeFromString()
bool PhysiologyEngineThunk::SerializeFromString | ( | std::string const & | state, |
std::string const & | data_requests, | ||
eSerializationFormat | format | ||
) |
◆ SerializeToFile()
bool PhysiologyEngineThunk::SerializeToFile | ( | std::string const & | filename | ) |
◆ SerializeToString()
std::string PhysiologyEngineThunk::SerializeToString | ( | eSerializationFormat | format | ) |
◆ SetEventHandler()
|
inline |
◆ SetLogFilename()
void PhysiologyEngineThunk::SetLogFilename | ( | std::string const & | logfile | ) |
◆ SetLoggerForward()
|
inline |
◆ SetupDefaultDataRequests()
|
protectedvirtual |
◆ SetupRequests()
|
protectedvirtual |
Member Data Documentation
◆ m_activeEvents
|
protected |
◆ m_dataDir
|
protected |
◆ m_engine
|
protected |
◆ m_events
|
protected |
◆ m_ForwardEvents
|
protected |
◆ m_ForwardLogs
|
protected |
◆ m_keepEventChanges
|
protected |
◆ m_keepLogMsgs
|
protected |
◆ m_length
|
protected |
◆ m_logMsgs
|
protected |
◆ m_requestedData
|
protected |
◆ m_requestedValues
|
protected |
◆ m_subMgr
|
protected |