Public Member Functions |
Protected Member Functions |
Protected Attributes |
Friends |
List of all members
SEPatientActionCollection Class Reference
#include <SEPatientActionCollection.h>
Inheritance diagram for SEPatientActionCollection:
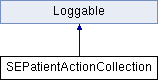
Protected Member Functions | |
SEPatientActionCollection (SESubstanceManager &subMgr) | |
void | Clear () |
bool | ProcessAction (const SEPatientAction &action) |
Friends | |
class | PBEngine |
class | SEActionManager |
Constructor & Destructor Documentation
◆ SEPatientActionCollection()
|
protected |
◆ ~SEPatientActionCollection()
SEPatientActionCollection::~SEPatientActionCollection | ( | ) |
Member Function Documentation
◆ Clear()
|
protected |
◆ GetAcuteRespiratoryDistressSyndromeExacerbation() [1/2]
SEAcuteRespiratoryDistressSyndromeExacerbation & SEPatientActionCollection::GetAcuteRespiratoryDistressSyndromeExacerbation | ( | ) |
◆ GetAcuteRespiratoryDistressSyndromeExacerbation() [2/2]
const SEAcuteRespiratoryDistressSyndromeExacerbation * SEPatientActionCollection::GetAcuteRespiratoryDistressSyndromeExacerbation | ( | ) | const |
◆ GetAcuteStress() [1/2]
SEAcuteStress & SEPatientActionCollection::GetAcuteStress | ( | ) |
◆ GetAcuteStress() [2/2]
const SEAcuteStress * SEPatientActionCollection::GetAcuteStress | ( | ) | const |
◆ GetAirwayObstruction() [1/2]
SEAirwayObstruction & SEPatientActionCollection::GetAirwayObstruction | ( | ) |
◆ GetAirwayObstruction() [2/2]
const SEAirwayObstruction * SEPatientActionCollection::GetAirwayObstruction | ( | ) | const |
◆ GetAllActions()
void SEPatientActionCollection::GetAllActions | ( | std::vector< const SEAction * > & | v | ) | const |
◆ GetArrhythmia() [1/2]
SEArrhythmia & SEPatientActionCollection::GetArrhythmia | ( | ) |
◆ GetArrhythmia() [2/2]
const SEArrhythmia * SEPatientActionCollection::GetArrhythmia | ( | ) | const |
◆ GetAsthmaAttack() [1/2]
SEAsthmaAttack & SEPatientActionCollection::GetAsthmaAttack | ( | ) |
◆ GetAsthmaAttack() [2/2]
const SEAsthmaAttack * SEPatientActionCollection::GetAsthmaAttack | ( | ) | const |
◆ GetBrainInjury() [1/2]
SEBrainInjury & SEPatientActionCollection::GetBrainInjury | ( | ) |
◆ GetBrainInjury() [2/2]
const SEBrainInjury * SEPatientActionCollection::GetBrainInjury | ( | ) | const |
◆ GetBronchoconstriction() [1/2]
SEBronchoconstriction & SEPatientActionCollection::GetBronchoconstriction | ( | ) |
◆ GetBronchoconstriction() [2/2]
const SEBronchoconstriction * SEPatientActionCollection::GetBronchoconstriction | ( | ) | const |
◆ GetCardiovascularMechanicsModification() [1/2]
SECardiovascularMechanicsModification & SEPatientActionCollection::GetCardiovascularMechanicsModification | ( | ) |
◆ GetCardiovascularMechanicsModification() [2/2]
const SECardiovascularMechanicsModification * SEPatientActionCollection::GetCardiovascularMechanicsModification | ( | ) | const |
◆ GetChestCompression() [1/2]
SEChestCompression & SEPatientActionCollection::GetChestCompression | ( | ) |
◆ GetChestCompression() [2/2]
const SEChestCompression * SEPatientActionCollection::GetChestCompression | ( | ) | const |
◆ GetChestCompressionAutomated() [1/2]
SEChestCompressionAutomated & SEPatientActionCollection::GetChestCompressionAutomated | ( | ) |
◆ GetChestCompressionAutomated() [2/2]
const SEChestCompressionAutomated * SEPatientActionCollection::GetChestCompressionAutomated | ( | ) | const |
◆ GetChestCompressionInstantaneous() [1/2]
SEChestCompressionInstantaneous & SEPatientActionCollection::GetChestCompressionInstantaneous | ( | ) |
◆ GetChestCompressionInstantaneous() [2/2]
const SEChestCompressionInstantaneous * SEPatientActionCollection::GetChestCompressionInstantaneous | ( | ) | const |
◆ GetChronicObstructivePulmonaryDiseaseExacerbation() [1/2]
SEChronicObstructivePulmonaryDiseaseExacerbation & SEPatientActionCollection::GetChronicObstructivePulmonaryDiseaseExacerbation | ( | ) |
◆ GetChronicObstructivePulmonaryDiseaseExacerbation() [2/2]
const SEChronicObstructivePulmonaryDiseaseExacerbation * SEPatientActionCollection::GetChronicObstructivePulmonaryDiseaseExacerbation | ( | ) | const |
◆ GetConsciousRespiration() [1/2]
SEConsciousRespiration & SEPatientActionCollection::GetConsciousRespiration | ( | ) |
◆ GetConsciousRespiration() [2/2]
const SEConsciousRespiration * SEPatientActionCollection::GetConsciousRespiration | ( | ) | const |
◆ GetConsumeNutrients() [1/2]
SEConsumeNutrients & SEPatientActionCollection::GetConsumeNutrients | ( | ) |
◆ GetConsumeNutrients() [2/2]
const SEConsumeNutrients * SEPatientActionCollection::GetConsumeNutrients | ( | ) | const |
◆ GetDyspnea() [1/2]
SEDyspnea & SEPatientActionCollection::GetDyspnea | ( | ) |
◆ GetDyspnea() [2/2]
const SEDyspnea * SEPatientActionCollection::GetDyspnea | ( | ) | const |
◆ GetExercise() [1/2]
SEExercise & SEPatientActionCollection::GetExercise | ( | ) |
◆ GetExercise() [2/2]
const SEExercise * SEPatientActionCollection::GetExercise | ( | ) | const |
◆ GetHemorrhage() [1/2]
SEHemorrhage & SEPatientActionCollection::GetHemorrhage | ( | eHemorrhage_Compartment | cmpt | ) |
◆ GetHemorrhage() [2/2]
const SEHemorrhage * SEPatientActionCollection::GetHemorrhage | ( | eHemorrhage_Compartment | cmpt | ) | const |
◆ GetHemorrhages() [1/2]
const std::vector< SEHemorrhage * > & SEPatientActionCollection::GetHemorrhages | ( | ) |
◆ GetHemorrhages() [2/2]
const std::vector< const SEHemorrhage * > SEPatientActionCollection::GetHemorrhages | ( | ) | const |
◆ GetImpairedAlveolarExchangeExacerbation() [1/2]
SEImpairedAlveolarExchangeExacerbation & SEPatientActionCollection::GetImpairedAlveolarExchangeExacerbation | ( | ) |
◆ GetImpairedAlveolarExchangeExacerbation() [2/2]
const SEImpairedAlveolarExchangeExacerbation * SEPatientActionCollection::GetImpairedAlveolarExchangeExacerbation | ( | ) | const |
◆ GetIntubation() [1/2]
SEIntubation & SEPatientActionCollection::GetIntubation | ( | ) |
◆ GetIntubation() [2/2]
const SEIntubation * SEPatientActionCollection::GetIntubation | ( | ) | const |
◆ GetLeftChestOcclusiveDressing() [1/2]
SEChestOcclusiveDressing & SEPatientActionCollection::GetLeftChestOcclusiveDressing | ( | ) |
◆ GetLeftChestOcclusiveDressing() [2/2]
const SEChestOcclusiveDressing * SEPatientActionCollection::GetLeftChestOcclusiveDressing | ( | ) | const |
◆ GetLeftClosedTensionPneumothorax() [1/2]
SETensionPneumothorax & SEPatientActionCollection::GetLeftClosedTensionPneumothorax | ( | ) |
◆ GetLeftClosedTensionPneumothorax() [2/2]
const SETensionPneumothorax * SEPatientActionCollection::GetLeftClosedTensionPneumothorax | ( | ) | const |
◆ GetLeftHemothorax() [1/2]
SEHemothorax & SEPatientActionCollection::GetLeftHemothorax | ( | ) |
◆ GetLeftHemothorax() [2/2]
const SEHemothorax * SEPatientActionCollection::GetLeftHemothorax | ( | ) | const |
◆ GetLeftNeedleDecompression() [1/2]
SENeedleDecompression & SEPatientActionCollection::GetLeftNeedleDecompression | ( | ) |
◆ GetLeftNeedleDecompression() [2/2]
const SENeedleDecompression * SEPatientActionCollection::GetLeftNeedleDecompression | ( | ) | const |
◆ GetLeftOpenTensionPneumothorax() [1/2]
SETensionPneumothorax & SEPatientActionCollection::GetLeftOpenTensionPneumothorax | ( | ) |
◆ GetLeftOpenTensionPneumothorax() [2/2]
const SETensionPneumothorax * SEPatientActionCollection::GetLeftOpenTensionPneumothorax | ( | ) | const |
◆ GetLeftTubeThoracostomy() [1/2]
SETubeThoracostomy & SEPatientActionCollection::GetLeftTubeThoracostomy | ( | ) |
◆ GetLeftTubeThoracostomy() [2/2]
const SETubeThoracostomy * SEPatientActionCollection::GetLeftTubeThoracostomy | ( | ) | const |
◆ GetMechanicalVentilation() [1/2]
SEMechanicalVentilation & SEPatientActionCollection::GetMechanicalVentilation | ( | ) |
◆ GetMechanicalVentilation() [2/2]
const SEMechanicalVentilation * SEPatientActionCollection::GetMechanicalVentilation | ( | ) | const |
◆ GetPericardialEffusion() [1/2]
SEPericardialEffusion & SEPatientActionCollection::GetPericardialEffusion | ( | ) |
◆ GetPericardialEffusion() [2/2]
const SEPericardialEffusion * SEPatientActionCollection::GetPericardialEffusion | ( | ) | const |
◆ GetPneumoniaExacerbation() [1/2]
SEPneumoniaExacerbation & SEPatientActionCollection::GetPneumoniaExacerbation | ( | ) |
◆ GetPneumoniaExacerbation() [2/2]
const SEPneumoniaExacerbation * SEPatientActionCollection::GetPneumoniaExacerbation | ( | ) | const |
◆ GetPulmonaryShuntExacerbation() [1/2]
SEPulmonaryShuntExacerbation & SEPatientActionCollection::GetPulmonaryShuntExacerbation | ( | ) |
◆ GetPulmonaryShuntExacerbation() [2/2]
const SEPulmonaryShuntExacerbation * SEPatientActionCollection::GetPulmonaryShuntExacerbation | ( | ) | const |
◆ GetRespiratoryFatigue() [1/2]
SERespiratoryFatigue & SEPatientActionCollection::GetRespiratoryFatigue | ( | ) |
◆ GetRespiratoryFatigue() [2/2]
const SERespiratoryFatigue * SEPatientActionCollection::GetRespiratoryFatigue | ( | ) | const |
◆ GetRespiratoryMechanicsConfiguration() [1/2]
SERespiratoryMechanicsConfiguration & SEPatientActionCollection::GetRespiratoryMechanicsConfiguration | ( | ) |
◆ GetRespiratoryMechanicsConfiguration() [2/2]
const SERespiratoryMechanicsConfiguration * SEPatientActionCollection::GetRespiratoryMechanicsConfiguration | ( | ) | const |
◆ GetRespiratoryMechanicsModification() [1/2]
SERespiratoryMechanicsModification & SEPatientActionCollection::GetRespiratoryMechanicsModification | ( | ) |
◆ GetRespiratoryMechanicsModification() [2/2]
const SERespiratoryMechanicsModification * SEPatientActionCollection::GetRespiratoryMechanicsModification | ( | ) | const |
◆ GetRightChestOcclusiveDressing() [1/2]
SEChestOcclusiveDressing & SEPatientActionCollection::GetRightChestOcclusiveDressing | ( | ) |
◆ GetRightChestOcclusiveDressing() [2/2]
const SEChestOcclusiveDressing * SEPatientActionCollection::GetRightChestOcclusiveDressing | ( | ) | const |
◆ GetRightClosedTensionPneumothorax() [1/2]
SETensionPneumothorax & SEPatientActionCollection::GetRightClosedTensionPneumothorax | ( | ) |
◆ GetRightClosedTensionPneumothorax() [2/2]
const SETensionPneumothorax * SEPatientActionCollection::GetRightClosedTensionPneumothorax | ( | ) | const |
◆ GetRightHemothorax() [1/2]
SEHemothorax & SEPatientActionCollection::GetRightHemothorax | ( | ) |
◆ GetRightHemothorax() [2/2]
const SEHemothorax * SEPatientActionCollection::GetRightHemothorax | ( | ) | const |
◆ GetRightNeedleDecompression() [1/2]
SENeedleDecompression & SEPatientActionCollection::GetRightNeedleDecompression | ( | ) |
◆ GetRightNeedleDecompression() [2/2]
const SENeedleDecompression * SEPatientActionCollection::GetRightNeedleDecompression | ( | ) | const |
◆ GetRightOpenTensionPneumothorax() [1/2]
SETensionPneumothorax & SEPatientActionCollection::GetRightOpenTensionPneumothorax | ( | ) |
◆ GetRightOpenTensionPneumothorax() [2/2]
const SETensionPneumothorax * SEPatientActionCollection::GetRightOpenTensionPneumothorax | ( | ) | const |
◆ GetRightTubeThoracostomy() [1/2]
SETubeThoracostomy & SEPatientActionCollection::GetRightTubeThoracostomy | ( | ) |
◆ GetRightTubeThoracostomy() [2/2]
const SETubeThoracostomy * SEPatientActionCollection::GetRightTubeThoracostomy | ( | ) | const |
◆ GetScalar()
const SEScalar * SEPatientActionCollection::GetScalar | ( | const std::string & | actionName, |
const std::string & | cmptName, | ||
const std::string & | substance, | ||
const std::string & | property | ||
) |
◆ GetSubstanceBolus() [1/2]
SESubstanceBolus & SEPatientActionCollection::GetSubstanceBolus | ( | const SESubstance & | sub | ) |
◆ GetSubstanceBolus() [2/2]
const SESubstanceBolus * SEPatientActionCollection::GetSubstanceBolus | ( | const SESubstance & | sub | ) | const |
◆ GetSubstanceBoluses() [1/2]
const std::vector< SESubstanceBolus * > & SEPatientActionCollection::GetSubstanceBoluses | ( | ) |
◆ GetSubstanceBoluses() [2/2]
const std::vector< const SESubstanceBolus * > SEPatientActionCollection::GetSubstanceBoluses | ( | ) | const |
◆ GetSubstanceCompoundInfusion() [1/2]
SESubstanceCompoundInfusion & SEPatientActionCollection::GetSubstanceCompoundInfusion | ( | const SESubstanceCompound & | sub | ) |
◆ GetSubstanceCompoundInfusion() [2/2]
const SESubstanceCompoundInfusion * SEPatientActionCollection::GetSubstanceCompoundInfusion | ( | const SESubstanceCompound & | sub | ) | const |
◆ GetSubstanceCompoundInfusions() [1/2]
const std::vector< SESubstanceCompoundInfusion * > & SEPatientActionCollection::GetSubstanceCompoundInfusions | ( | ) |
◆ GetSubstanceCompoundInfusions() [2/2]
const std::vector< const SESubstanceCompoundInfusion * > SEPatientActionCollection::GetSubstanceCompoundInfusions | ( | ) | const |
◆ GetSubstanceInfusion() [1/2]
SESubstanceInfusion & SEPatientActionCollection::GetSubstanceInfusion | ( | const SESubstance & | sub | ) |
◆ GetSubstanceInfusion() [2/2]
const SESubstanceInfusion * SEPatientActionCollection::GetSubstanceInfusion | ( | const SESubstance & | sub | ) | const |
◆ GetSubstanceInfusions() [1/2]
const std::vector< SESubstanceInfusion * > & SEPatientActionCollection::GetSubstanceInfusions | ( | ) |
◆ GetSubstanceInfusions() [2/2]
const std::vector< const SESubstanceInfusion * > SEPatientActionCollection::GetSubstanceInfusions | ( | ) | const |
◆ GetSupplementalOxygen() [1/2]
SESupplementalOxygen & SEPatientActionCollection::GetSupplementalOxygen | ( | ) |
◆ GetSupplementalOxygen() [2/2]
const SESupplementalOxygen * SEPatientActionCollection::GetSupplementalOxygen | ( | ) | const |
◆ GetUrinate() [1/2]
SEUrinate & SEPatientActionCollection::GetUrinate | ( | ) |
◆ GetUrinate() [2/2]
const SEUrinate * SEPatientActionCollection::GetUrinate | ( | ) | const |
◆ HasActiveCPRAction()
bool SEPatientActionCollection::HasActiveCPRAction | ( | ) | const |
◆ HasAcuteRespiratoryDistressSyndromeExacerbation()
bool SEPatientActionCollection::HasAcuteRespiratoryDistressSyndromeExacerbation | ( | ) | const |
◆ HasAcuteStress()
bool SEPatientActionCollection::HasAcuteStress | ( | ) | const |
◆ HasAirwayObstruction()
bool SEPatientActionCollection::HasAirwayObstruction | ( | ) | const |
◆ HasArrhythmia()
bool SEPatientActionCollection::HasArrhythmia | ( | ) | const |
◆ HasAsthmaAttack()
bool SEPatientActionCollection::HasAsthmaAttack | ( | ) | const |
◆ HasBrainInjury()
bool SEPatientActionCollection::HasBrainInjury | ( | ) | const |
◆ HasBronchoconstriction()
bool SEPatientActionCollection::HasBronchoconstriction | ( | ) | const |
◆ HasCardiovascularMechanicsModification()
bool SEPatientActionCollection::HasCardiovascularMechanicsModification | ( | ) | const |
◆ HasChestCompression()
bool SEPatientActionCollection::HasChestCompression | ( | ) | const |
◆ HasChestCompressionAutomated()
bool SEPatientActionCollection::HasChestCompressionAutomated | ( | ) | const |
◆ HasChestCompressionInstantaneous()
bool SEPatientActionCollection::HasChestCompressionInstantaneous | ( | ) | const |
◆ HasChestOcclusiveDressing()
bool SEPatientActionCollection::HasChestOcclusiveDressing | ( | ) | const |
◆ HasChronicObstructivePulmonaryDiseaseExacerbation()
bool SEPatientActionCollection::HasChronicObstructivePulmonaryDiseaseExacerbation | ( | ) | const |
◆ HasConsciousRespiration()
bool SEPatientActionCollection::HasConsciousRespiration | ( | ) | const |
◆ HasConsumeNutrients()
bool SEPatientActionCollection::HasConsumeNutrients | ( | ) | const |
◆ HasDyspnea()
bool SEPatientActionCollection::HasDyspnea | ( | ) | const |
◆ HasExercise()
bool SEPatientActionCollection::HasExercise | ( | ) | const |
◆ HasHemorrhage() [1/2]
bool SEPatientActionCollection::HasHemorrhage | ( | ) | const |
◆ HasHemorrhage() [2/2]
bool SEPatientActionCollection::HasHemorrhage | ( | eHemorrhage_Compartment | cmpt | ) | const |
◆ HasHemothorax()
bool SEPatientActionCollection::HasHemothorax | ( | ) | const |
◆ HasImpairedAlveolarExchangeExacerbation()
bool SEPatientActionCollection::HasImpairedAlveolarExchangeExacerbation | ( | ) | const |
◆ HasIntubation()
bool SEPatientActionCollection::HasIntubation | ( | ) | const |
◆ HasLeftChestOcclusiveDressing()
bool SEPatientActionCollection::HasLeftChestOcclusiveDressing | ( | ) | const |
◆ HasLeftClosedTensionPneumothorax()
bool SEPatientActionCollection::HasLeftClosedTensionPneumothorax | ( | ) | const |
◆ HasLeftHemothorax()
bool SEPatientActionCollection::HasLeftHemothorax | ( | ) | const |
◆ HasLeftNeedleDecompression()
bool SEPatientActionCollection::HasLeftNeedleDecompression | ( | ) | const |
◆ HasLeftOpenTensionPneumothorax()
bool SEPatientActionCollection::HasLeftOpenTensionPneumothorax | ( | ) | const |
◆ HasLeftTubeThoracostomy()
bool SEPatientActionCollection::HasLeftTubeThoracostomy | ( | ) | const |
◆ HasMechanicalVentilation()
bool SEPatientActionCollection::HasMechanicalVentilation | ( | ) | const |
◆ HasNeedleDecompression()
bool SEPatientActionCollection::HasNeedleDecompression | ( | ) | const |
◆ HasPericardialEffusion()
bool SEPatientActionCollection::HasPericardialEffusion | ( | ) | const |
◆ HasPneumoniaExacerbation()
bool SEPatientActionCollection::HasPneumoniaExacerbation | ( | ) | const |
◆ HasPulmonaryShuntExacerbation()
bool SEPatientActionCollection::HasPulmonaryShuntExacerbation | ( | ) | const |
◆ HasRespiratoryFatigue()
bool SEPatientActionCollection::HasRespiratoryFatigue | ( | ) | const |
◆ HasRespiratoryMechanicsConfiguration()
bool SEPatientActionCollection::HasRespiratoryMechanicsConfiguration | ( | ) | const |
◆ HasRespiratoryMechanicsModification()
bool SEPatientActionCollection::HasRespiratoryMechanicsModification | ( | ) | const |
◆ HasRightChestOcclusiveDressing()
bool SEPatientActionCollection::HasRightChestOcclusiveDressing | ( | ) | const |
◆ HasRightClosedTensionPneumothorax()
bool SEPatientActionCollection::HasRightClosedTensionPneumothorax | ( | ) | const |
◆ HasRightHemothorax()
bool SEPatientActionCollection::HasRightHemothorax | ( | ) | const |
◆ HasRightNeedleDecompression()
bool SEPatientActionCollection::HasRightNeedleDecompression | ( | ) | const |
◆ HasRightOpenTensionPneumothorax()
bool SEPatientActionCollection::HasRightOpenTensionPneumothorax | ( | ) | const |
◆ HasRightTubeThoracostomy()
bool SEPatientActionCollection::HasRightTubeThoracostomy | ( | ) | const |
◆ HasSubstanceBolus() [1/2]
bool SEPatientActionCollection::HasSubstanceBolus | ( | ) | const |
◆ HasSubstanceBolus() [2/2]
bool SEPatientActionCollection::HasSubstanceBolus | ( | const SESubstance & | sub | ) | const |
◆ HasSubstanceCompoundInfusion() [1/2]
bool SEPatientActionCollection::HasSubstanceCompoundInfusion | ( | ) | const |
◆ HasSubstanceCompoundInfusion() [2/2]
bool SEPatientActionCollection::HasSubstanceCompoundInfusion | ( | const SESubstanceCompound & | sub | ) | const |
◆ HasSubstanceInfusion() [1/2]
bool SEPatientActionCollection::HasSubstanceInfusion | ( | ) | const |
◆ HasSubstanceInfusion() [2/2]
bool SEPatientActionCollection::HasSubstanceInfusion | ( | const SESubstance & | sub | ) | const |
◆ HasSupplementalOxygen()
bool SEPatientActionCollection::HasSupplementalOxygen | ( | ) | const |
◆ HasTensionPneumothorax()
bool SEPatientActionCollection::HasTensionPneumothorax | ( | ) | const |
◆ HasTubeThoracostomy()
bool SEPatientActionCollection::HasTubeThoracostomy | ( | ) | const |
◆ HasUrinate()
bool SEPatientActionCollection::HasUrinate | ( | ) | const |
◆ ProcessAction()
|
protected |
◆ RemoveAcuteRespiratoryDistressSyndromeExacerbation()
void SEPatientActionCollection::RemoveAcuteRespiratoryDistressSyndromeExacerbation | ( | ) |
◆ RemoveAcuteStress()
void SEPatientActionCollection::RemoveAcuteStress | ( | ) |
◆ RemoveAirwayObstruction()
void SEPatientActionCollection::RemoveAirwayObstruction | ( | ) |
◆ RemoveArrhythmia()
void SEPatientActionCollection::RemoveArrhythmia | ( | ) |
◆ RemoveAsthmaAttack()
void SEPatientActionCollection::RemoveAsthmaAttack | ( | ) |
◆ RemoveBrainInjury()
void SEPatientActionCollection::RemoveBrainInjury | ( | ) |
◆ RemoveBronchoconstriction()
void SEPatientActionCollection::RemoveBronchoconstriction | ( | ) |
◆ RemoveCardiovascularMechanicsModification()
void SEPatientActionCollection::RemoveCardiovascularMechanicsModification | ( | ) |
◆ RemoveChestCompression()
void SEPatientActionCollection::RemoveChestCompression | ( | ) |
◆ RemoveChestCompressionAutomated()
void SEPatientActionCollection::RemoveChestCompressionAutomated | ( | ) |
◆ RemoveChestCompressionInstantaneous()
void SEPatientActionCollection::RemoveChestCompressionInstantaneous | ( | ) |
◆ RemoveChronicObstructivePulmonaryDiseaseExacerbation()
void SEPatientActionCollection::RemoveChronicObstructivePulmonaryDiseaseExacerbation | ( | ) |
◆ RemoveConsciousRespiration()
void SEPatientActionCollection::RemoveConsciousRespiration | ( | ) |
◆ RemoveConsumeNutrients()
void SEPatientActionCollection::RemoveConsumeNutrients | ( | ) |
◆ RemoveDyspnea()
void SEPatientActionCollection::RemoveDyspnea | ( | ) |
◆ RemoveExercise()
void SEPatientActionCollection::RemoveExercise | ( | ) |
◆ RemoveHemorrhage()
void SEPatientActionCollection::RemoveHemorrhage | ( | eHemorrhage_Compartment | cmpt | ) |
◆ RemoveImpairedAlveolarExchangeExacerbation()
void SEPatientActionCollection::RemoveImpairedAlveolarExchangeExacerbation | ( | ) |
◆ RemoveIntubation()
void SEPatientActionCollection::RemoveIntubation | ( | ) |
◆ RemoveLeftChestOcclusiveDressing()
void SEPatientActionCollection::RemoveLeftChestOcclusiveDressing | ( | ) |
◆ RemoveLeftClosedTensionPneumothorax()
void SEPatientActionCollection::RemoveLeftClosedTensionPneumothorax | ( | ) |
◆ RemoveLeftHemothorax()
void SEPatientActionCollection::RemoveLeftHemothorax | ( | ) |
◆ RemoveLeftNeedleDecompression()
void SEPatientActionCollection::RemoveLeftNeedleDecompression | ( | ) |
◆ RemoveLeftOpenTensionPneumothorax()
void SEPatientActionCollection::RemoveLeftOpenTensionPneumothorax | ( | ) |
◆ RemoveLeftTubeThoracostomy()
void SEPatientActionCollection::RemoveLeftTubeThoracostomy | ( | ) |
◆ RemoveMechanicalVentilation()
void SEPatientActionCollection::RemoveMechanicalVentilation | ( | ) |
◆ RemovePericardialEffusion()
void SEPatientActionCollection::RemovePericardialEffusion | ( | ) |
◆ RemovePneumoniaExacerbation()
void SEPatientActionCollection::RemovePneumoniaExacerbation | ( | ) |
◆ RemovePulmonaryShuntExacerbation()
void SEPatientActionCollection::RemovePulmonaryShuntExacerbation | ( | ) |
◆ RemoveRespiratoryFatigue()
void SEPatientActionCollection::RemoveRespiratoryFatigue | ( | ) |
◆ RemoveRespiratoryMechanicsConfiguration()
void SEPatientActionCollection::RemoveRespiratoryMechanicsConfiguration | ( | ) |
◆ RemoveRespiratoryMechanicsModification()
void SEPatientActionCollection::RemoveRespiratoryMechanicsModification | ( | ) |
◆ RemoveRightChestOcclusiveDressing()
void SEPatientActionCollection::RemoveRightChestOcclusiveDressing | ( | ) |
◆ RemoveRightClosedTensionPneumothorax()
void SEPatientActionCollection::RemoveRightClosedTensionPneumothorax | ( | ) |
◆ RemoveRightHemothorax()
void SEPatientActionCollection::RemoveRightHemothorax | ( | ) |
◆ RemoveRightNeedleDecompression()
void SEPatientActionCollection::RemoveRightNeedleDecompression | ( | ) |
◆ RemoveRightOpenTensionPneumothorax()
void SEPatientActionCollection::RemoveRightOpenTensionPneumothorax | ( | ) |
◆ RemoveRightTubeThoracostomy()
void SEPatientActionCollection::RemoveRightTubeThoracostomy | ( | ) |
◆ RemoveSubstanceBolus()
void SEPatientActionCollection::RemoveSubstanceBolus | ( | const SESubstance & | sub | ) |
◆ RemoveSubstanceCompoundInfusion()
void SEPatientActionCollection::RemoveSubstanceCompoundInfusion | ( | const SESubstanceCompound & | sub | ) |
◆ RemoveSubstanceInfusion()
void SEPatientActionCollection::RemoveSubstanceInfusion | ( | const SESubstance & | sub | ) |
◆ RemoveSupplementalOxygen()
void SEPatientActionCollection::RemoveSupplementalOxygen | ( | ) |
◆ RemoveUrinate()
void SEPatientActionCollection::RemoveUrinate | ( | ) |
Friends And Related Function Documentation
◆ PBEngine
|
friend |
◆ SEActionManager
|
friend |
Member Data Documentation
◆ m_AcuteStress
|
protected |
◆ m_AirwayObstruction
|
protected |
◆ m_ARDSExacerbation
|
protected |
◆ m_Arrhythmia
|
protected |
◆ m_AsthmaAttack
|
protected |
◆ m_BrainInjury
|
protected |
◆ m_Bronchoconstriction
|
protected |
◆ m_CardiovascularMechanicsModification
|
protected |
◆ m_ChestCompression
|
protected |
◆ m_ChestCompressionAutomated
|
protected |
◆ m_ChestCompressionInstantaneous
|
protected |
◆ m_ConsciousRespiration
|
protected |
◆ m_ConsumeNutrients
|
protected |
◆ m_COPDExacerbation
|
protected |
◆ m_Dyspnea
|
protected |
◆ m_Exercise
|
protected |
◆ m_Hemorrhages
|
protected |
◆ m_ImpairedAlveolarExchangeExacerbation
|
protected |
◆ m_Intubation
|
protected |
◆ m_LeftChestOcclusiveDressing
|
protected |
◆ m_LeftClosedTensionPneumothorax
|
protected |
◆ m_LeftHemothorax
|
protected |
◆ m_LeftNeedleDecompression
|
protected |
◆ m_LeftOpenTensionPneumothorax
|
protected |
◆ m_LeftTubeThoracostomy
|
protected |
◆ m_MechanicalVentilation
|
protected |
◆ m_PericardialEffusion
|
protected |
◆ m_PneumoniaExacerbation
|
protected |
◆ m_PulmonaryShuntExacerbation
|
protected |
◆ m_RespiratoryFatigue
|
protected |
◆ m_RespiratoryMechanicsConfiguration
|
protected |
◆ m_RespiratoryMechanicsModification
|
protected |
◆ m_RightChestOcclusiveDressing
|
protected |
◆ m_RightClosedTensionPneumothorax
|
protected |
◆ m_RightHemothorax
|
protected |
◆ m_RightNeedleDecompression
|
protected |
◆ m_RightOpenTensionPneumothorax
|
protected |
◆ m_RightTubeThoracostomy
|
protected |
◆ m_SubMgr
|
protected |
◆ m_SubstanceBoluses
|
protected |
◆ m_SubstanceCompoundInfusions
|
protected |
◆ m_SubstanceInfusions
|
protected |
◆ m_SupplementalOxygen
|
protected |
◆ m_Urinate
|
protected |